Deluge or Data Enriched Language for the Universal Grid Environment is an online scripting language integrated with Zoho Creator. It helps you to add different types of business logic to the function and make it more powerful. The entire database layer is abstracted and you will only map the arguments with fields, while scripting in Deluge. See Also Deluge Script - Reference Guide
Target Audience
- CRM Users with Manage Automation permissions
- Programmers with Deluge Script knowledge
Availability
Profile Permission Required: Manage Automation
Program Functions
Programming function through Deluge Script includes the following three steps:
- Program a function.
- Associate function to a workflow rule.
- Test the function integration.
To program a function
- Go to Setup > Automation > Actions > function.
- In the Workflow Functions page, click the Configure function.
- In the Configure function page, click the Write your own function link.
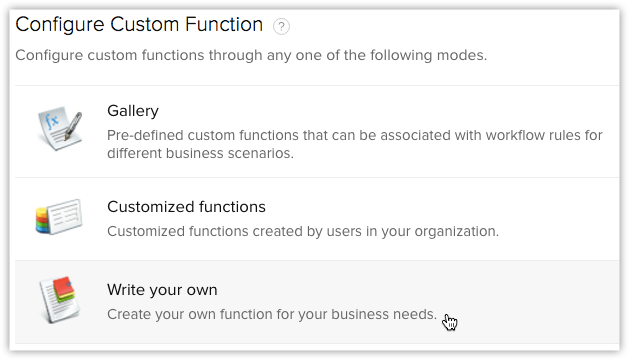
- In the Customized functions page, complete the following:
- Specify name and description of the function.
- Choose the module to be associated with the function and click the Edit Arguments link.
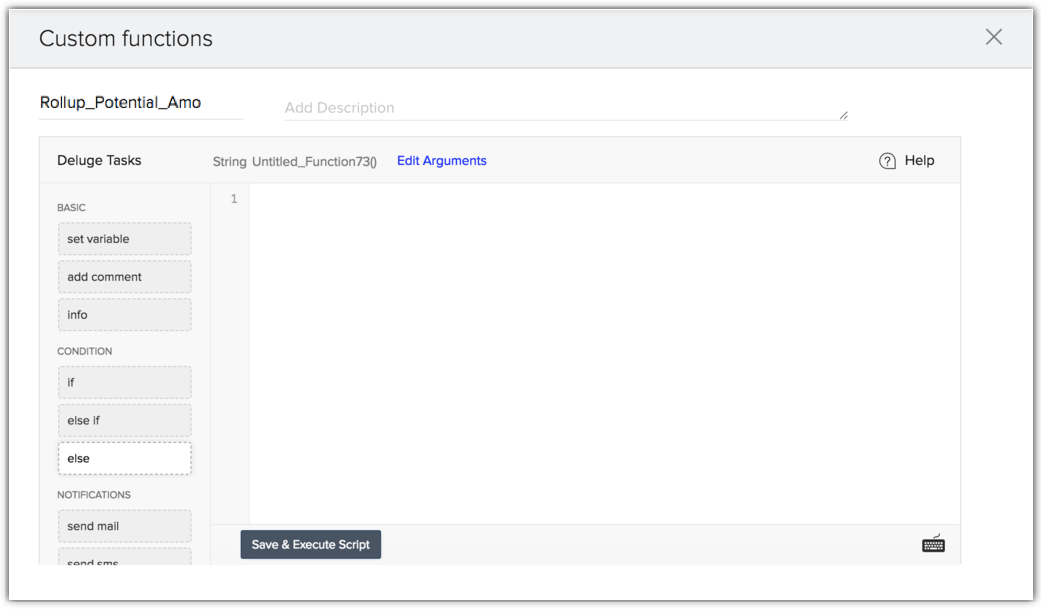
- Now map the argument with field name or custom value in CRM module.
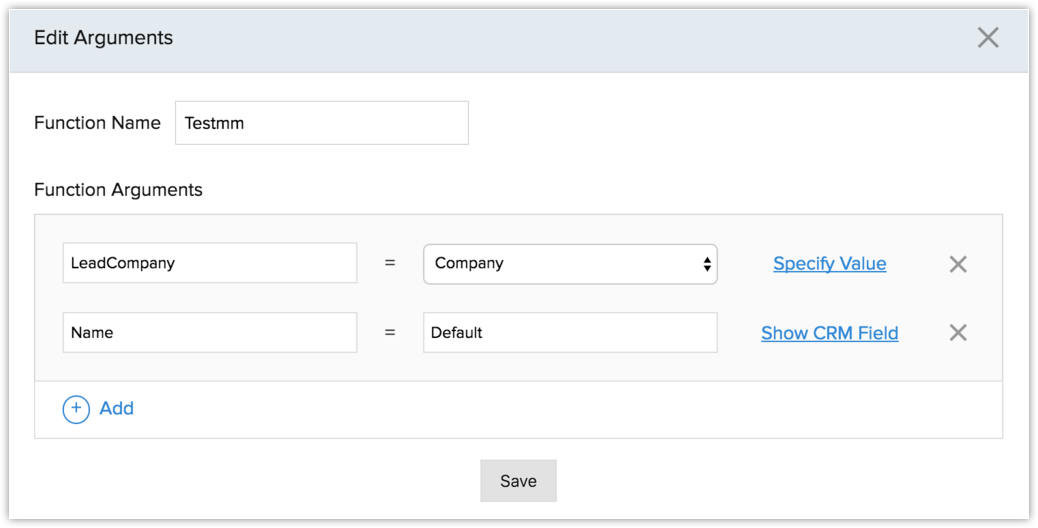
- Click Done.
- Write your required function in Deluge Script Editor. Click Save & Execute Script to validate your code.
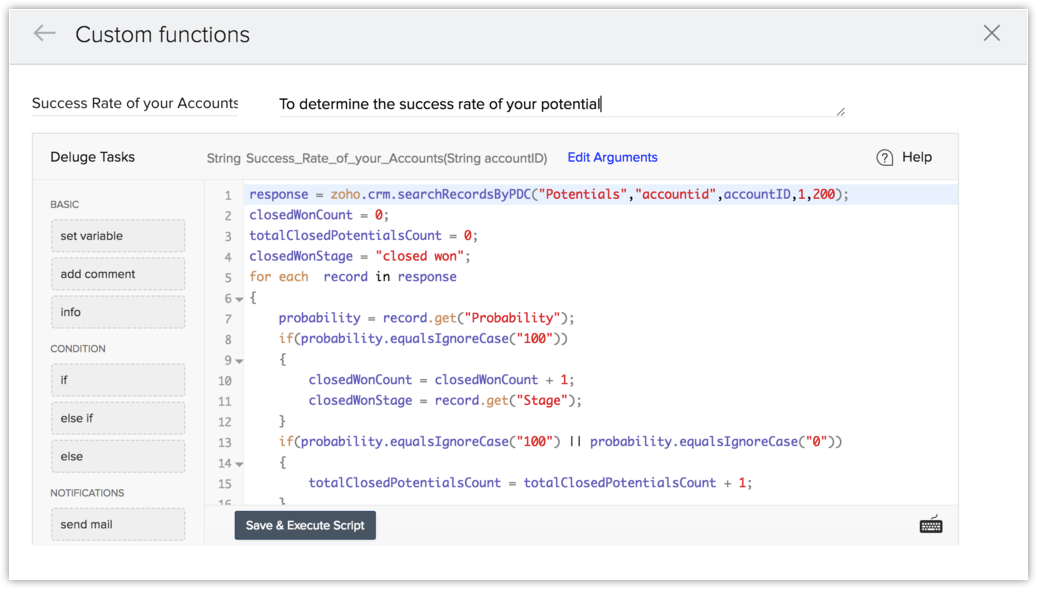
If there are no errors, your script is ready to be associated with the Workflow Rule.
- Click Save.
To associate function to a workflow rule
- Go to Setup > Automation > Workflow Rules.
- In the Workflow Rules page, click the Create Rule.
- In the Create New Rule tab, specify workflow rule parameters.
- Select function, under Instant Actions.
- Now select the function to be triggered.
- Click Save.
To test the function integration
- Add test data in CRM according to your workflow rule criteria.
- In your application check for the data received from Zoho CRM via function.
- If there is an error or data mismatch, modify your function code in Zoho CRM.
- Continue this test until you obtain the required data from Zoho CRM to your Application.
Important Notes
- You can associate up to 6 (1 Instant Action and 5 Time-Based Actions) Functions per workflow rule.
- You can transfer data for a maximum of 10 CRM fields from Zoho CRM to third-party applications using function.
- You can retrieve data from other Apps to Zoho CRM using function.
- You must update the API ticket regularly according to limits in third-party applications.
- You will not receive any email notification, if the function integration stopped functioning due to any issue in a third-party API.
- If there is any failure in the process, function will send a notification first, Zoho CRM system will send a second notification after 15 min. Thereafter, the system will not send any notifications for that particular workflow trigger.
- If you exceed the maximum count per day, the system will not send remaining function notifications to third-party applications and will notify thw failure to Administrator.
- Zoho CRM will the send the data in following standard format for Date and Date Time fields to third-party applications:
- Date: yyyy-MM-dd
- Date Time: yyyy-MM-dd hh:mm
Understanding Deluge Script Builder
The Deluge Script Builder helps you to program the Functions using drag & drop user interface and execute the function within CRM. It enables you to convert the complex application logic into simple deluge code, without remembering the syntax and functions of Deluge Script. It contains the following components:
- Delug Script Code
- Deluge Tasks
- Conditions
- Miscellaneous
- Debug
- List Manipulation
- Map Manipulation
- Web Data
- XML Manipulation
- Code Execution
List of Deluge Tasks
Example
Business Scenario: Roll up the amount field to parent account when the potential status is "closed won"
Summary
Roll-up amount from each potential (status is closed won) to Total Revenue (custom field) field of the related Account. If customer buys 10 times, you can view the total revenue from Account page, instead of pulling a report linking Potentials and Accounts. Based on the revenue from customer, later you can grade them type A, type B, or type C customers.
Programming Steps
void workflowspace.Rollup_the_Potential_Amount_to_Accounts(int AccountId, string SumUpField)
- void is the return type that will return nothing
- workflowspace is the namespace in the creator just like a folder to group functions
- Rollup_the_Potential_Amount_to_Accounts is the name of the function
- AccountId is a parameter, which holds the ID of Account in CRM
- SumUpField is another parameter, which holds the field label in Account to which the sum amount is updated while triggering the workflow rule.
{ accountID1 = (input.AccountId).toString();
- Here we are type casting account id to a string
resp = zoho.crm.searchRecordsByPDC("Potentials", ("accountid"), accountID1);
- This is a built in method in Deluge Script, which will get the records by searching the field values.
- Here we specified to search rgw Potential record type which matches the Account Id with the value that is passed as a parameter.
- Initialising a local variable to calculate the sum of amount.
- Iterating all potentials returned by the above method call.
{
stage = (rec.get("Stage")).toLowerCase();
- Fetching the stage field value of each potential.
if (stage.contains("won"))
- Checking the stage value contains "won" for each potential. If it is true, then it will enter to the next line, otherwise go to the next potential.
{
total = (total + (rec.get("Amount")).toDecimal());
- Now adding the amount field's value to the local variable
} }
updateResp = zoho.crm.updateRecord(("Accounts"), accountID1, { input.SumUpField : total });
}
- This method will update the record in CRM. We mentioned the record type as Account matching the field account id with the passed value with the local variable, which currently holds the sum up value.
Complete Program
void workflowspace.Rollup_the_Potential_Amount_to_Accounts(int AccountId, string SumUpField)
{
accountID1 = (input.AccountId).toString();
resp = zoho.crm.searchRecordsByPDC("Potentials", ("accountid"), accountID1);
info resp;
total = 0.0;
for each rec in resp
{
stage = (rec.get("Stage")).toLowerCase();
if (stage.contains("won"))
{
total = (total + (rec.get("Amount")).toDecimal());
}
}
updateResp = zoho.crm.updateRecord(("Accounts"), accountID1, { input.SumUpField : total });
}
Follow these steps to configure the function:
- Log in to Zoho CRM.
- Go to Setup > Automation > Workflow Rules.
- Select Deals from the Module drop-down. Enter the name and description to the workflow rule.
- Choose when you want to execute this rule. Select Create or Edit option under execute based on a record action.
- Click Done.
- Choose to which records you want to execute the rule. Select Records Matcing the conditon option and set the criteria as "Stage contains closed won"
- Click Done.
- Now choose function as Instant action
- In the Configure function pop-up, click the Customized Functions link.
- In the Customized Functions page, click Configure for Roll-up Potential Amount to Account function.
- Select Potential as your module.
- Specify values for the arguments as mentioned below:
- AccountId: Choose "AccountId" column from the list of fields.
- SumUpField: Specify the field you have in accounts module for the summation.
- Click "Specify custom value" link and type your field name.
- Save the configuration. This will now automatically update the sum of the revenue generated by all the potentials of an account in the account module.